[TOC]
### **WebSocket接口配置说明**
选中组件(本文以百分比条形图为例说明),在操作界面右侧,点击图标,并选择WebSocket,如下图
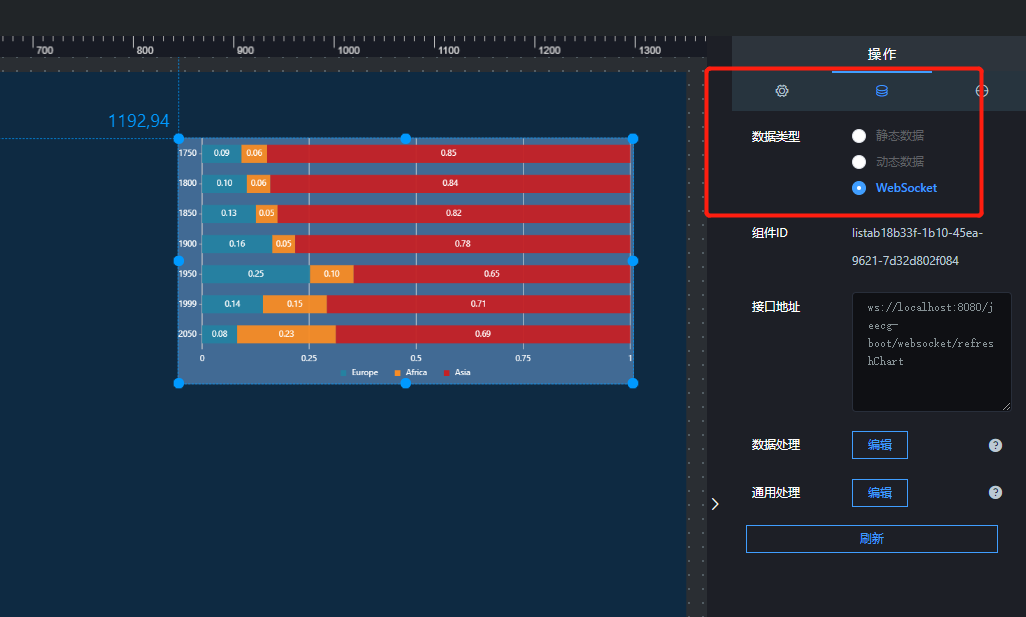
### **1、配置接口地址**
在接口地址一栏填写我们制作的接口地址,并点击刷新应用接口,如下图
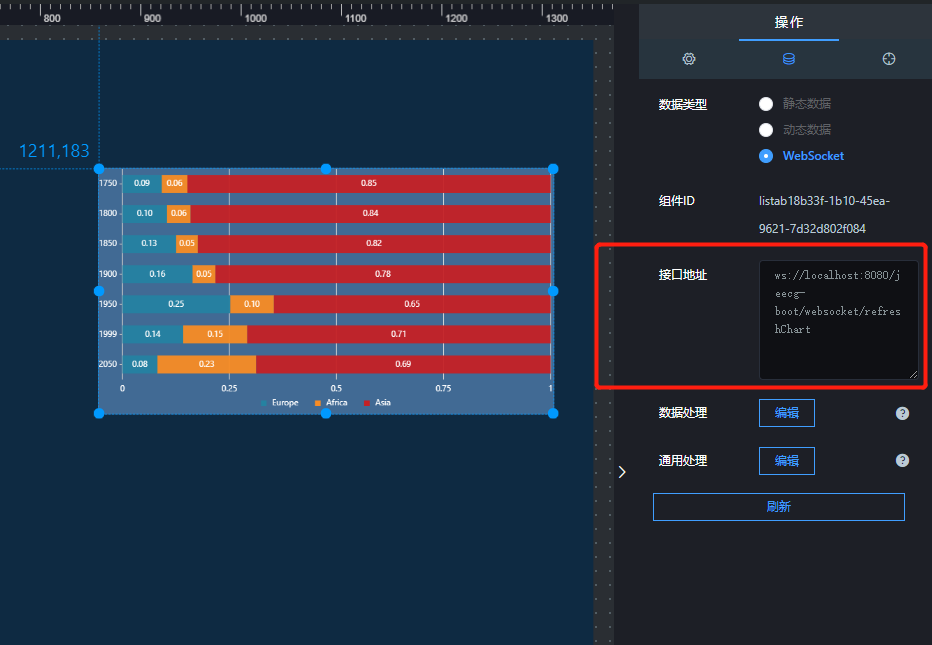
接口地址示例:ws://localhost:8080/jeecg-boot/websocket/refreshChart
https使用wss
### **2、编写WebSocket服务端**
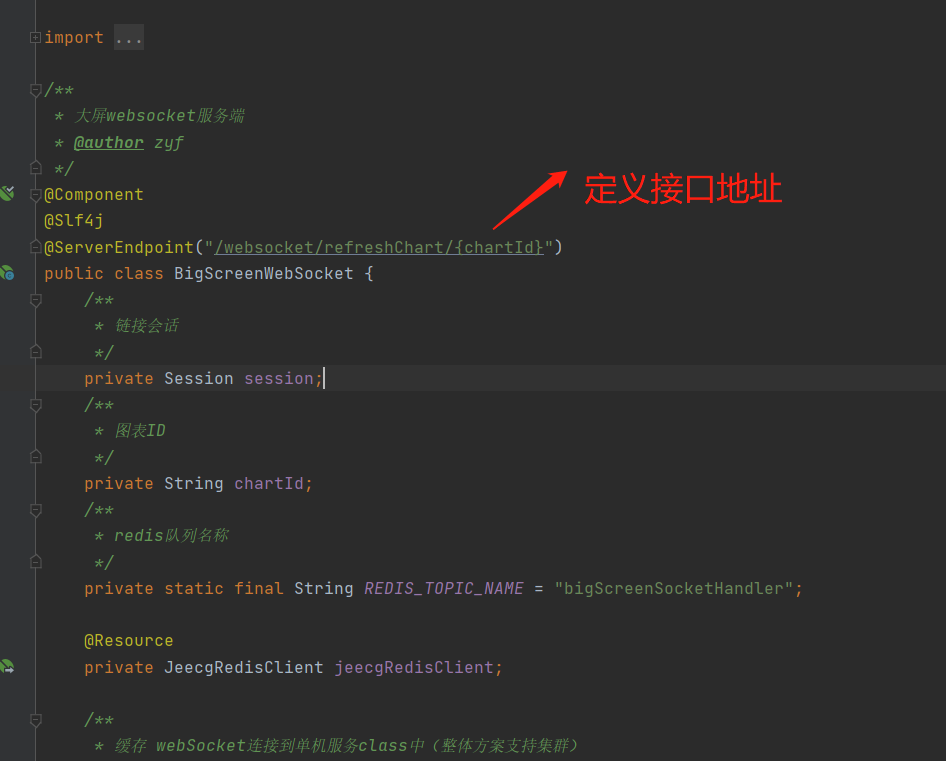
示例代码如下:
示例中使用了([redis订阅发布](https://blog.csdn.net/qq_39507276/article/details/89045275))机制解决消息集群问题
```
package org.jeecg.modules.jmreport.visual.websocket;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.CopyOnWriteArraySet;
import javax.annotation.Resource;
import javax.websocket.OnClose;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.PathParam;
import javax.websocket.server.ServerEndpoint;
import org.jeecg.common.base.BaseMap;
import org.jeecg.common.constant.WebsocketConst;
import org.jeecg.common.modules.redis.client.JeecgRedisClient;
import org.springframework.stereotype.Component;
import com.alibaba.fastjson.JSONObject;
import lombok.extern.slf4j.Slf4j;
/**
* 大屏websocket服务端
* @author zyf
*/
@Component
@Slf4j
@ServerEndpoint("/websocket/refreshChart/{chartId}")
public class BigScreenWebSocket {
/**
* 链接会话
*/
private Session session;
/**
* 图表ID
*/
private String chartId;
/**
* redis队列名称
*/
private static final String REDIS_TOPIC_NAME = "bigScreenSocketHandler";
@Resource
private JeecgRedisClient jeecgRedisClient;
/**
* 缓存 webSocket连接到单机服务class中(整体方案支持集群)
*/
private static CopyOnWriteArraySet<BigScreenWebSocket> webSockets = new CopyOnWriteArraySet<>();
private static Map<String, Session> sessionPool = new HashMap<String, Session>();
@OnOpen
public void onOpen(Session session, @PathParam(value = "chartId") String chartId) {
try {
this.session = session;
this.chartId = chartId;
webSockets.add(this);
sessionPool.put(chartId, session);
log.info("【websocket消息】有新的连接,总数为:" + webSockets.size());
} catch (Exception e) {
}
}
@OnClose
public void onClose() {
try {
webSockets.remove(this);
sessionPool.remove(this.chartId);
log.info("【websocket消息】连接断开,总数为:" + webSockets.size());
} catch (Exception e) {
}
}
/**
* 服务端推送消息
*
* @param chartId
* @param message
*/
public void pushMessage(String chartId, String message) {
Session session = sessionPool.get(chartId);
if (session != null && session.isOpen()) {
try {
log.info("【websocket消息】 单点消息:" + message);
session.getAsyncRemote().sendText(message);
} catch (Exception e) {
e.printStackTrace();
}
}
}
/**
* 服务器端推送消息
*/
public void pushMessage(String message) {
try {
webSockets.forEach(ws -> ws.session.getAsyncRemote().sendText(message));
} catch (Exception e) {
e.printStackTrace();
}
}
@OnMessage
public void onMessage(String message) {
//todo 现在有个定时任务刷,应该去掉
log.debug("【websocket消息】收到客户端消息:" + message);
JSONObject obj = new JSONObject();
//业务类型
obj.put(WebsocketConst.MSG_CMD, WebsocketConst.CMD_CHECK);
//消息内容
obj.put(WebsocketConst.MSG_TXT, "心跳响应");
for (BigScreenWebSocket webSocket : webSockets) {
webSocket.pushMessage(message);
}
}
/**
* 后台发送消息到redis
*
* @param message
*/
public void sendMessage(String message) {
log.info("【websocket消息】广播消息:" + message);
BaseMap baseMap = new BaseMap();
baseMap.put("chartId", "");
baseMap.put("message", message);
jeecgRedisClient.sendMessage(REDIS_TOPIC_NAME, baseMap);
}
/**
* 此为单点消息
*
* @param chartId
* @param message
*/
public void sendMessage(String chartId, String message) {
BaseMap baseMap = new BaseMap();
baseMap.put("chartId", chartId);
baseMap.put("message", message);
jeecgRedisClient.sendMessage(REDIS_TOPIC_NAME, baseMap);
}
/**
* 此为单点消息(多组件)
*
* @param chartIds
* @param message
*/
public void sendMessage(String[] chartIds, String message) {
for (String chartId : chartIds) {
sendMessage(chartId, message);
}
}
}
```
### **3、编写消息推送测试方法**
```
/**
* 测试大屏websocket接口
*/
@RestController
@Api(tags="大屏WebSocket测试")
@RequestMapping("/bigscreen/websocket")
public class WebSocketController {
@Autowired
private BigScreenWebSocket webSocket;
@PostMapping("/sendData")
@ApiOperation(value="测试大屏组件更新", notes="测试大屏组件更新")
public Result<String> sendData() {
Result<String> result = new Result<String>();
//需要推送数据的组件ID
String chartId="listab18b33f-1b10-45ea-9621-7d32d802f084";
String message = "[{\"country\":\"Asia\",\"year\":\"1750\",\"value\":1502},{\"country\":\"Asia\",\"year\":\"1800\",\"value\":1635},{\"country\":\"Asia\",\"year\":\"1850\",\"value\":1809},{\"country\":\"Asia\",\"year\":\"1900\",\"value\":1947},{\"country\":\"Asia\",\"year\":\"1950\",\"value\":1402},{\"country\":\"Asia\",\"year\":\"1999\",\"value\":3634},{\"country\":\"Asia\",\"year\":\"2050\",\"value\":5268},{\"country\":\"Africa\",\"year\":\"1750\",\"value\":106},{\"country\":\"Africa\",\"year\":\"1800\",\"value\":107},{\"country\":\"Africa\",\"year\":\"1850\",\"value\":111},{\"country\":\"Africa\",\"year\":\"1900\",\"value\":133},{\"country\":\"Africa\",\"year\":\"1950\",\"value\":221},{\"country\":\"Africa\",\"year\":\"1999\",\"value\":767},{\"country\":\"Africa\",\"year\":\"2050\",\"value\":1766},{\"country\":\"Europe\",\"year\":\"1750\",\"value\":163},{\"country\":\"Europe\",\"year\":\"1800\",\"value\":203},{\"country\":\"Europe\",\"year\":\"1850\",\"value\":276},{\"country\":\"Europe\",\"year\":\"1900\",\"value\":408},{\"country\":\"Europe\",\"year\":\"1950\",\"value\":547},{\"country\":\"Europe\",\"year\":\"1999\",\"value\":729},{\"country\":\"Europe\",\"year\":\"2050\",\"value\":628}]";
JSONObject obj = new JSONObject();
obj.put("chartId",chartId);
obj.put("result", message);
webSocket.sendMessage(chartId, obj.toJSONString());
result.setResult("单发");
return result;
}
}
```
### **4、返回JSON数据格式**
```
[{
"value": 1048,
"name": "波导33333"
},
{
"value": 735,
"name": "oppo"
},
{
"value": 580,
"name": "华为"
},
{
"value": 484,
"name": "小米"
},
{
"value": 300,
"name": "魅族"
}]
```
- 项目介绍
- 常见问题
- 积木报表版本升级注意事项
- 集成文档
- 快速集成
- Docker部署
- 示例Demo
- 项目集成
- springboot集成积木报表
- JeecgBoot集成积木报表
- 如何配置访问菜单
- ruoyi单体版集成积木报表
- ruoyi vue版集成积木报表
- eladmin集成积木报表
- guns集成积木报表
- docker-compose部署
- 旧版本
- 快速集成1.4.3
- 快速集成1.0.1
- 快速集成1.2
- 示例demo1.2
- minidao版本出炉
- Maven私服
- 常见问题bak
- 升级说明
- jeecgcloud集成积木报表
- 快速集成1.8.1
- 开发配置
- 数据库兼容
- yaml配置参数说明
- 文件上传配置
- 系统上下文变量
- Token权限控制
- 云存储跨域设置
- 连接池参数配置
- 分库数据源配置
- 微服务下静态资源访问不到
- 多租户配置
- 快速入门
- 1. 整体页面布局介绍
- 2. SQL数据源报表制作
- 3. SQL数据源报表带参制作
- 4. API数据源报表制作
- 5. API数据源报表带参制作
- 6. 如何从0到1创建一个大屏
- 7.API接口数据格式适配
- 8.api数据集token机制详解
- 9.api数据集打印全部规则说明
- 10.自定义api接口数据格式转换器
- 11.数据库支持类型
- 12、数据源用法介绍
- 错误使用数据集示例
- 操作手册
- 报表设计器
- 一、基础操作
- 1 登录
- 2. 创建报表
- 3. 编辑报表
- 4. 背景设置
- 5. 复制、粘贴、剪切
- 6. 行操作
- 7. 添加数据源
- 8. 报表数据集
- 8.1 SQL数据集配置
- 8.2 API数据集配置
- 8.3 JavaBean数据集
- 8.4 对象数据集配置
- 8.5 集合数据集配置
- 8.6 JSON数据集配置
- 8.7 sql数据源解析失败弹窗
- 8.8 存储过程
- 8.9 Redis数据集
- 8.10 MongoDB数据集
- 9. 插入数据
- 10. 数据格式化设置
- 11. 数据字典配置
- 12. 导入报表
- 13. 导出报表
- 14. 分页设置
- 15. 报表集成
- 16. 分享报表
- 17.报表模版库
- 18.数据源查询默认值配置
- 19.自定义分页条数
- 20.报表定时保存配置
- 21.补全空白行
- 22.预览页工具条设置
- 23.动态合并格
- 24.图片设置
- 二、打印设计
- 1.打印设置规则_重要
- 2. 打印区域设置
- 3. 打印清晰度设置
- 4. 打印机样式设置
- 5. 套打怎样设置
- 6. 带背景打印
- 三、数据报表设计
- 1. 分组
- 1.1 纵向分组(相邻合并)
- 1.2 横向分组(相邻合并)
- 1.3 横向动态列分组
- 1.4 横向纵向组合动态列分组
- 1.5 横向分组小计
- 1.6 分组内合计
- 1.7 纵向分组小计
- 1.8 常用分组示例
- 1.9 分组数据排序设置
- 2. 表达式(旧)
- 3.查询条件
- 3.1 API查询条件配置
- 3.2 API查询条件为时间
- 3.3 API自定义查询条件
- 3.4 SQL配置查询条件
- 3.5 API接口后台接收参数说明
- 3.6 API范围查询
- 4.条形码/二维码
- 4.1 二维码配置说明
- 4.2 条形码配置说明
- 4.3 条形码和二维码改值
- 5.交叉报表
- 6.明细报表
- 7.主子报表
- 7.1 主子表API数据源(新)
- 7.2 主子表SQL数据源(新)
- 7.3 主子表SQL关联(旧)
- 8.联动钻取
- 8.1 报表联动(新)
- 8.2 报表钻取(新)
- 8.3 报表钻取带条件用法
- 8.4 图表钻取带条件用法
- 8.5 报表联动图表带条件用法
- 8.6 图表联动图表带条件用法
- 8.7 报表钻取(旧)
- 8.8 报表联动(旧)
- 8.9 钻取联动条件规则
- 9.组件交互清单
- 10.循环块设置
- 11.分栏设置
- 12.分版设置
- 13多表头复杂报表
- 四、表达式函数
- 数据集表达式
- 单元格表达式
- 统计函数
- 行号函数
- 日期函数
- 数学函数
- 字符串函数
- 条件表达式
- 颜色表达式
- 判断函数
- 函数库列表(高级)
- 基本运算符(高级)
- 自定义报表函数
- 五、报表查询配置
- 报表参数配置
- 报表查询配置
- 查询控件类型
- 查询控件默认值
- 时间控件设置说明
- 时间控件默认值设置
- SQL中条件表达式
- 查询控件下拉树
- 范围查询设置默认值
- JS增强和CSS增强
- 技巧说明
- 参数公用示例
- 六、图形报表设计
- 1. 基本配置
- 1.1 图表背景设置
- 1.2 图表动态刷新
- 1.3 图表之间的联动
- 2. 柱形图配置
- 数据源配置
- a)单数据源配置
- b)多数据源配置
- 3. 折线图配置
- 数据源配置
- a)单数据源配置
- b)多数据源配置
- 4. 饼图配置
- 数据源配置
- 5. 折柱图配置
- 数据源配置
- 6. 散点图配置
- 6.1 普通散点图数据源配置
- 6.2 气泡散点图数据源配置
- 7. 漏斗图配置
- 数据源配置
- 8. 象形图配置
- 数据源配置
- 9. 地图配置
- 地图区配置
- 数据源配置
- 10. 仪表盘配置
- 数据源配置
- 11. 雷达图配置
- 数据源配置
- 12. 关系图配置
- 数据源
- 七、专项功能小结
- sql数据集 in查询
- 联动/钻取配置说明
- 数据集字段字典配置
- 传参渲染到报表上
- 测试超Z列效果
- Issue提问指南
- 钻取、联动条件用法
- 导出图片支持背景导出
- 模板示例补充表
- 暂取消功能
- Pdf导出接口方案
- ExceL导出接口方案
- pdf浏览器打印
- 报表分组设计
- 纵向分组
- 纵向分组说明
- 横向自定义分组
- 组合动态列分组
- 纵向多维分组
- 横向多维分组
- 横向组内小计
- 纵向分组小计
- 大屏设计器
- 大屏常见问题
- 一、基础操作
- 1. 登录
- 2. 新建大屏
- 3.修改大屏
- 4. 预览、保存
- 5. 画布介绍
- 6. 添加组件
- 7. 组件图层位置
- 8. 其他操作
- 9. 大屏集成
- 10. 组合分组
- 11、大屏属性配置
- 二、组件配置说明
- 1 通用配置
- 1.1 图层名称
- 1.2 标题配置
- 1.3 X轴配置
- 1.4 Y轴配置
- 1.5 提示语设置
- 1.6 数值设置
- 1.7 坐标轴边距设置
- 1.8 图例设置
- 1.9 自定义配色
- 1.10 系统配色
- 1.11 动画设置
- 2 图表类组件
- 2.1 柱形图
- 数据格式
- 数据格式(新)
- 2.2 堆叠柱形图
- 数据格式
- 2.3 胶囊图
- 数据格式
- 2.4 玉珏图
- 数据格式
- 2.5 折线图
- 数据格式
- 数据格式(新)
- 2.6 饼图
- 数据格式
- 2.7 mini环形图(已删)
- 数据格式
- 2.8 动态环图
- 数据格式
- 2.9 象形柱图
- 数据格式
- 2.9 象形图
- 数据格式
- 2.10 雷达图
- 数据格式
- 2.11 散点图
- 2.11.1 数据源配置
- 数据格式 (新)
- 2.12 漏斗图
- 数据格式
- 2.13 折柱图
- 数据格式
- 2.14 多色仪表盘
- 数据格式
- 2.15 水波图
- 数据格式
- 2.16 环形图
- 数据格式
- 2.18 仪盘表
- 数据格式
- 2.19 金字塔漏斗图
- 数据格式
- 2.20 旋转饼图
- 数据格式
- 2.21 子弹图
- 数据格式
- 2.22 气泡图
- 数据格式
- 2.23 男女占比
- 数据格式
- 2.24 对称条形图
- 数据格式
- 2.25 百分比条形图
- 数据格式
- 3 文本类组件
- 3.1 文本框
- 3.2 跑马灯
- 3.3 超链接(旧)
- 3.4 实时时间
- 3.5 翻牌器
- 数据格式
- 3.6 字符云
- 数据格式
- 3.7 图层字符云
- 数据格式
- 3.8 闪动字符云
- 数据格式
- 3.9 天气预报
- 3.10 颜色块
- 数据格式
- 4 装饰类组件
- 4.1 图片组件
- 数据格式
- 4.2 边框
- 4.3 装饰
- 5 视频类组件
- 5.1 video
- 数据格式
- 5.2 RTMP 播放器
- 5.3 阿里播放器
- 6 表格类组件
- 6.1 排名表
- 数据格式
- 6.2 轮播表
- 数据格式
- 6.3 表格
- 数据格式
- 6.4 个性排名
- 数据格式
- 6.5 气泡排名
- 数据格式
- 6.6 发展历程
- 数据格式
- 7 地图类组件
- 离线地图
- 销量排名地图
- 数据格式
- 气泡标注地图
- 数据格式
- 综合统计地图
- 数据格式
- 飞线地图
- 数据格式
- 全国地图
- 数据格式
- 城市派件地图
- 数据格式
- 联网地图
- 全国地图
- 数据格式
- 飞线图
- 数据格式
- 热力图
- 数据格式
- 柱形地图
- 数据格式
- 标注地图
- 数据格式
- 百度空气质量图
- 数据格式
- 8 其他组件
- 8.1 选项卡
- 数据格式
- 8.2 轮播图
- 数据格式
- 8.3 iframe
- 数据格式
- 9 万能组件
- 9.1 堆叠条形图
- 9.2 正负条形图
- 9.3 双向对比柱形图
- 9.4 圆形柱形图
- 9.5 嵌套饼图
- 9.6 矩形树图
- 9.7 k线图
- 10 高级扩展
- 通用处理
- 数据处理
- 提示事件
- 标题事件
- 11 交互配置
- 组件联动
- 组件钻取
- 区域点击
- 12 数据源配置
- 静态数据
- 动态数据
- WebSocket接口
- 接口参数
- sql数据源
- 三、系统集成
- 1. 大屏访问Token安全方案
- 仪表盘/门户设计器
- 新功能20221008
- 打印支持回调接口
- 打印支持设定表头表尾
- 自动换行改造说明